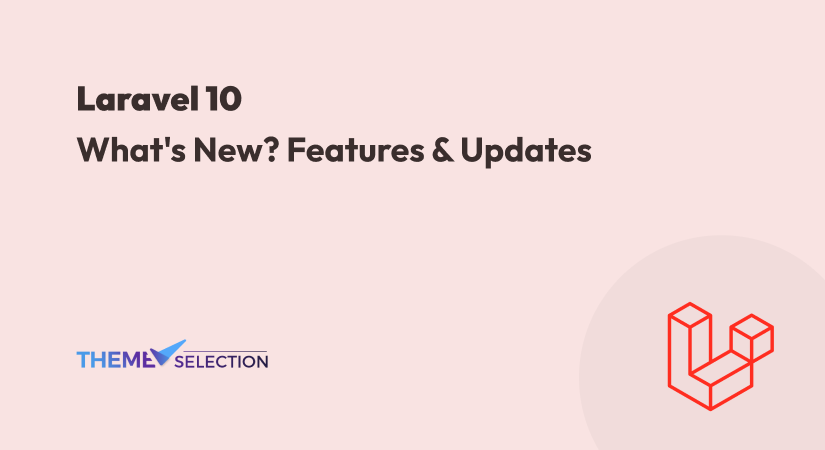
Laravel 10 is here with some awesome updates & some changes..!! Well, it continues the improvements made in Laravel 9.x by introducing argument and return types to all application skeleton methods, as well as all stub files used to generate classes throughout the framework.
In addition, there is a new developer-friendly abstraction layer for starting and interacting with external processes. Furthermore, Laravel Pennant has been introduced to provide a great approach to managing your application’s “feature flags”.
We will discuss here some of the major changes that have been brought to the Laravel 10
Table of contents
Laravel Release Schedule
So, the Laravel team used to release two big updates a year, but they changed things up and now release just one major version annually. This lets them really focus on making each new version awesome without causing any issues.
They released Laravel 9 on February 8, 2022, and were planning on putting out Laravel 10 on February 7, 2023, but they needed some extra time to perfect it. So, it actually came out on February 14, 2023. Next up is Laravel 11, which should be available in the first part of February 2024.
Oh, and just so you know, according to the support policy Laravel will provide bug fixes for 18 months and security updates for two years for all their versions. Here’s when you can expect those updates for each version:
The following are the expected bug fixes and security updates schedule:
- Laravel 9 will get bug fixes until August 8, 2023, and security fixes until February 6, 2024.
- Laravel 10 will receive bug fixes and security updates until August 6, 2024, and February 4, 2025.
- For Laravel 11, Bug fixes and security updates will be available until August 5, 2025, and February 3, 2026, respectively.
It is advisable to use the developer-friendly & highly customizable Laravel Admin Template for your upcoming project for better results.
Now, let’s check what’s new in Laravel 10..!!
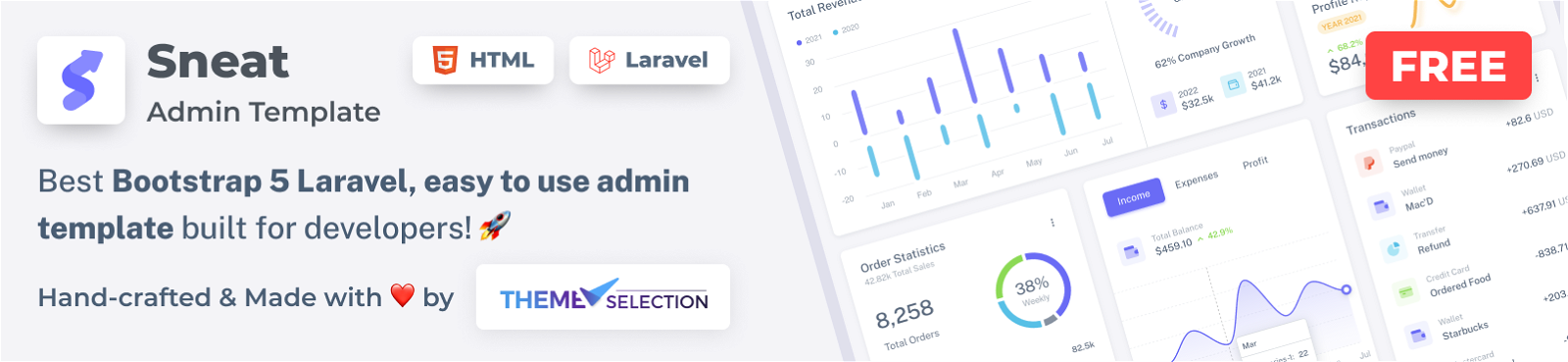
What’s New In Laravel 10?
There’s no doubt that the most exciting part about any new release is the addition of new features. So without further ado, let’s start by looking at the new features and updates in Laravel 10.
Following are the Major Updates:
Laravel 10 Support for PHP 8.1 & 8.2
In Laravel 10, the minimum required version of PHP has been updated to PHP 8.1, with some of its features, such as read-only properties and array_is_list, being used within the framework. It’s worth noting that support for PHP 8.0 has been dropped.
Although PHP 8.2 was only released two months before Laravel 10, there’s no need to worry about compatibility issues. Laravel 10 is fully prepared to work with PHP 8.2 without requiring any additional adjustments.
In fact, the entire Laravel ecosystem, including Forge, Vapor, and Envoyer, fully supports PHP 8.2. Additionally, PHP 8.2 is also compatible with Laravel 9, offering you more flexibility in your development choices.
Here are the features PHP 8.2 will include:
- Consistent data classes
- Use of nested attributes
- Native support to Enums
- First-class Callable syntax
- No overridden in child classes
- Explicit Octal numeral notation
- Use of string keys to unpack arrays
- Intersection types satisfy multiple constraints at the same time
Laravel Pennant
The Laravel Pennant package has been released as a first-party package. Using Laravel Pennant, you can manage your application’s feature flags in a lightweight, streamlined manner. In addition to an in-memory array driver, Pennant includes a database driver for the persistent storage of features.
As a result of Pennant’s advanced features and APIs, it offers a wide range of capabilities. Please refer to the comprehensive Pennant documentation for more information.
Features can be easily defined via the Feature::define method:
use Laravel\Pennant\Feature;
use Illuminate\Support\Lottery;
Feature::define('new-onboarding-flow', function () {
return Lottery::odds(1, 10);
});
Once a feature has been defined, you may easily determine if the current user has access to the given feature:
if (Feature::active('new-onboarding-flow')) {
// ...
}
Laravel Process Interaction
The new Process Interactions in Laravel 10 make testing and running CLI processes a piece of the cake. It offers a straightforward API to ease the burden of testing
Laravel 10.x introduces a beautiful abstraction layer for starting and interacting with external processes via a new Process
facade:
use Illuminate\Support\Facades\Process;
$result = Process::run('ls -la');
return $result->output();
The process may even start in pools, which will allow convenient execution and concurrent process management:
use Illuminate\Process\Pool;
use Illuminate\Support\Facades\Process;
[$first, $second, $third] = Process::concurrently(function (Pool $pool) {
$pool->command('cat first.txt');
$pool->command('cat second.txt');
$pool->command('cat third.txt');
});
return $first->output();
It can be even faked for convenient testing:
Process::fake();
// ...
Process::assertRan('ls -la');
For further details check the documentation.
Test Profiling – Identify Slow Running Test
The Artisantest
command has received a new <strong>--profile</strong>
option that allows you to easily identify the slowest tests in your application:
php artisan test --profile
For convenience, the slowest tests will be displayed directly within the CLI output:
The processing functionality in Laravel is designed to cater to the most frequent usage scenarios, resulting in an exceptional experience for developers.
Predis Version Upgrade
Predis is a powerful Redis client for PHP that can significantly enhance caching capabilities and improve user experience. In earlier versions, Laravel supported both Predis 1 and 2. However, Laravel 10 has discontinued support for Predis 1.
While Laravel documentation recommends using Predis as the package for interacting with Redis, you may also choose to utilize the official PHP extension. This extension offers an API for communicating with Redis servers and can be used as an alternative to Predis.
Pest Scaffolding
Pest is a lightweight testing framework that simplifies the testing process and makes it more readable and enjoyable. With Pest scaffolding, developers can now generate Pest tests and use them out-of-the-box without any additional configuration.
Laravel 10 now includes pest test scaffolding as a default feature when creating new projects. You can enable this feature using the <strong>--pest flag</strong>
when building your application with the Laravel installer:
laravel new example-materio-admin--pest
Native Type Declaration:
Laravel 10 introduces native type declarations in the application skeleton code, allowing for type-hinting and return types in any code generated by the framework. This change provides several benefits for developers, including better type clarity and enhanced IDE support for parameter and response shapes.
Types are being added in a way that brings the latest PHP type-hinting features to Laravel projects without breaking backward compatibility at the framework level:
- Return types
- Method arguments
- Redundant annotations are removed where possible
- Allow user land types in closure arguments
- Does not include typed properties
Overall, the addition of native-type declarations in Laravel 10 enhances the developer experience and facilitates more efficient and maintainable code. It provides better type safety and allows IDEs to perform better with auto-complete features.
While there are some caveats to this approach, we believe that developers will appreciate the benefits of this new feature when creating new projects in the future.
Method Signature + Return Types
In its initial release, Laravel utilized all the available type-hinting features in PHP. However, as additional features such as return types, union types, and more primitive type-hints were introduced in PHP, Laravel has incorporated them to provide a more robust developer experience.
Laravel 10.x introduces a comprehensive update to the application skeleton and all stubs used by the framework, adding argument and return types to all method signatures. Furthermore, redundant “doc block” type-hint information has been removed to provide better code clarity.
It is important to note that this change is entirely backward compatible with existing applications. Applications that do not utilize these type-hints will continue to function normally. Overall, this update enhances the developer experience by providing better type safety and facilitating more efficient and maintainable code.
Invokable Validation Rules
In Laravel 9, you had to add the –invokable flag to the Artisan command to create an invokable validation rule. However, this extra step is no longer required in Laravel 10 as all rules are invokable by default, which demonstrates the streamlined process for creating invokable validation rules in Laravel 10. Check the PR on GitHub.
So, you may run the following command to create a new invokable rule in Laravel 10:
php artisan make: rule CustomRule
Furthermore, Artisan in Laravel 10 now includes improved error handling for missing model names. If Artisan cannot find a specified model name, it will prompt the user to provide one and also ask if they want to create a factory, migration, or other related items. This enhancement streamlines the development process by reducing the likelihood of errors and providing a more user-friendly experience.
Hashing Algorithm Is Now Faster Than Ever
xxHash is a lightning-fast hash algorithm that boasts exceptional output randomness, dispersion, and uniqueness to minimize collisions. PHP 8.1 has extended support for xxh128, making it an ideal hash algorithm for Laravel 10, which runs on PHP 8.1.
It’s worth noting that Taylor has cautioned that some third-party packages may rely on file names being in the exact format as the SHA-1 hash, which was Laravel’s previous hashing algorithm. Hence, if you’re planning to upgrade to Laravel 10, it’s recommended to carefully review any third-party packages utilized in your app to ensure they support xxHash.
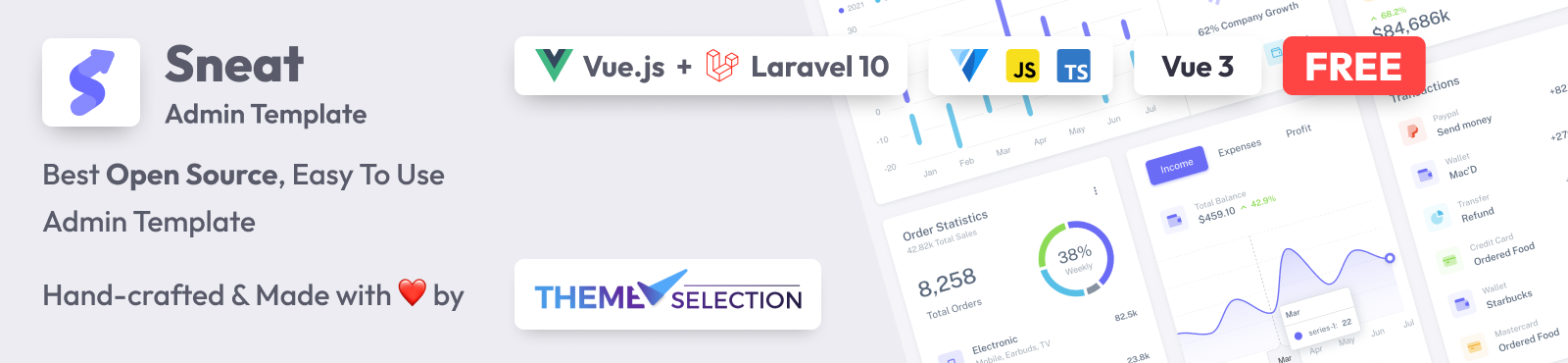
Removed dispatchNow()
In Laravel 10, the ‘dispatchNow()’ method has been removed as it was deprecated in Laravel 9 in favor of ‘dispatchSync()’. It is essential to replace it in all your existing projects, as it constitutes a breaking change, but the solution is simple.
Column Type Native Retrieval
Laravel 10 introduces a significant feature that eliminates the need to depend on the doctrine/dbal package when using the <strong>Schema::getColumnType</strong>
method. This feature enables direct data type conversion by the database drivers, resulting in faster data processing and reduced conversions.
In addition to improved performance, the native retrieval feature allows you to write integration tests for the new column modifying feature. By using this feature, you can obtain the data type name or the entire type definition of a specified column.
Laravel Official Packages Upgrade
Not only is the framework professionally maintained and updated on a regular basis, but so are all of the official packages and the ecosystem.
The following is a list of the most recent official Laravel packages that have been updated to support Laravel 10:
- Breeze
- Cashier Stripe
- Dusk
- Horizon
- Installer
- Jetstream
- Passport
- Pint
- Sail
- Scout
- Valet
Deprecations from Laravel 9
Methods marked as deprecated in Laravel 9 are being removed in Laravel 10. We can expect the release upgrade guide to outline all the deprecated methods, potential impact assessment, and how to upgrade closer to the release.
Here are some deprecations found in the comparison of the Laravel framework’s master branch to the 9.x branch at the time of writing:
- Remove various deprecations Pull Request #41136
- Remove deprecated dates property in Pull Request #42587
- Remove handleDeprecation the method in Pull Request #42590
- Remove deprecated assertTimesSent method Pull Request #42592
- Remove deprecated ScheduleListCommand‘s $defaultName property 419471e
- Remove deprecated Route::home method Pull Request #42614
- Remove deprecated dispatchNow functionality Pull Request #42591
Should You Upgrade to Laravel 10?
When considering upgrading an application’s Laravel version, it’s essential to weigh the benefits against the potential risks and disruptions. While Laravel is an open-source framework, each instance installed on a machine has a unique codebase, and upgrading to a new version requires careful consideration of the impact on the existing codebase.
It’s worth noting that using an unsupported version of the framework doesn’t necessarily mean that the application will stop working, but it may pose security risks and require more maintenance effort. Therefore, it’s generally recommended to prioritize application stability over framework upgrades and only upgrade to the latest version after thorough testing and careful planning.
In short, you should consider upgrading to Laravel 10 when:
- The application is stable with its current version and functioning without problems.
- The new version either adds a feature that your application requires or fixes an issue that your application is experiencing.
- The application will be well-tested before the upgrade changes are pushed into production.
How Can I Upgrade To Laravel 10?
If you are considering upgrading to Laravel 10, the good news is that the Laravel core team has put considerable effort into providing a comprehensive upgrade guide that covers all possible breaking changes, ensuring a seamless and straightforward transition. You can find the official Laravel 10 upgrade guide to help you with the process.
In addition, Laravel Shift is also a valuable tool to consider now that Laravel 10 has been released. This automated platform provides a simple and efficient approach to upgrading your Laravel version. The process is as easy as deploying Laravel 9, making it a convenient option for developers.
To run Laravel 10, it is essential to have the following server requirements:
- PHP >= 8.1
- Ctype PHP Extension
- cURL PHP Extension
- DOM PHP Extension
- Fileinfo PHP Extension
- Filter PHP Extension
- Hash PHP Extension
- Mbstring PHP Extension
- OpenSSL PHP Extension
- PCRE PHP Extension
- PDO PHP Extension
- Session PHP Extension
- Tokenizer PHP Extension
- XML PHP Extension
As said above, do consider your application requirement first.
Conclusion:
Well, here we talked about some of the major changes that have been brought with the latest Laravel 10 update. The Laravel team has been very keen when it comes to bringing changes that helps make it easy to work with laravel. They also take care of the fact that changes should not affect the previous development. Developers can still work with laravel 9 and they have enough time to migrate to laravel 10.
You may have questions regarding the future as well. As discussed above, you can expect changes every 6 months and a new version i.e. Laravel 1 1 in 2024. We can expect many other exciting changes in the upcoming version as they have made some awesome changes to Laravel 10.
We assure you we will keep this blog updated for you. Whenever there is a new update, we will include it in this blog. So, bookmark this blog and keep an eye on it for upcoming updates.